Pagination with SSG
This example shows how to implement page based pagination with SSG in Next.js.
The first 5 paginated pages are cached in the edge at build time, and the rest are incrementally cached using ISR, that way we can avoid increasing build times no matter how many pages we have while still keeping essential pages cached from the start.
The example showcases a PLP (Product Listing Pages) where:
- There are 100 test products and 1 category (PLP)
- There are 10 results per page for a total of 10 pages, where 5 are pre-generated with
getStaticPaths
// pages/category/[page].tsx export const getStaticPaths = async () => { return { // Prerender the next 5 pages after the first page, which is handled by the index page. // Other pages will be prerendered at runtime. paths: Array.from({ length: 5 }).map((_, i) => `/category/${i + 2}`), // Block the request for non-generated pages and cache them in the background fallback: 'blocking', } }
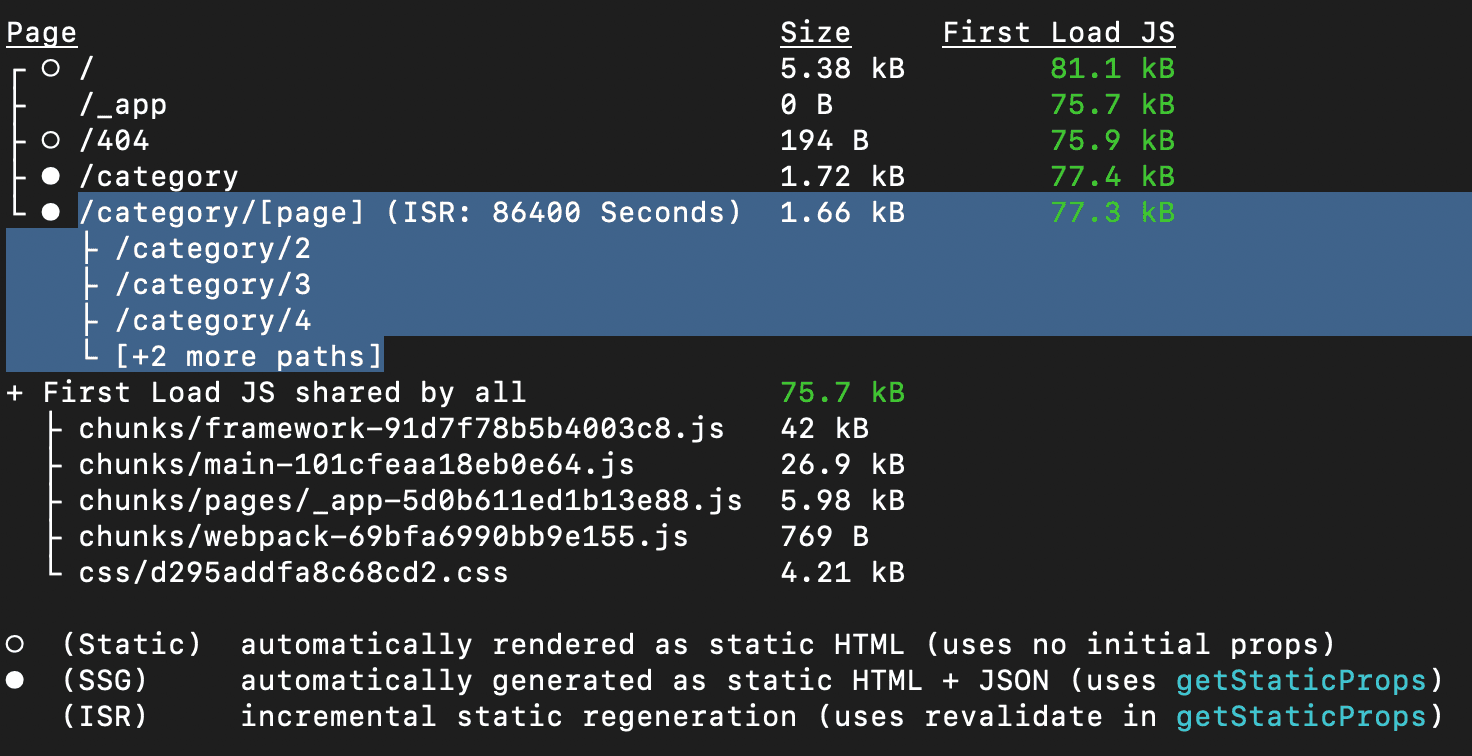